In the world of web development, building high-performance APIs is a must. As more users and requests flood in, optimizing your API’s performance becomes crucial. Spring, a popular Java framework, offers a powerful solution by introducing asynchronous programming using @Async and CompletableFuture.
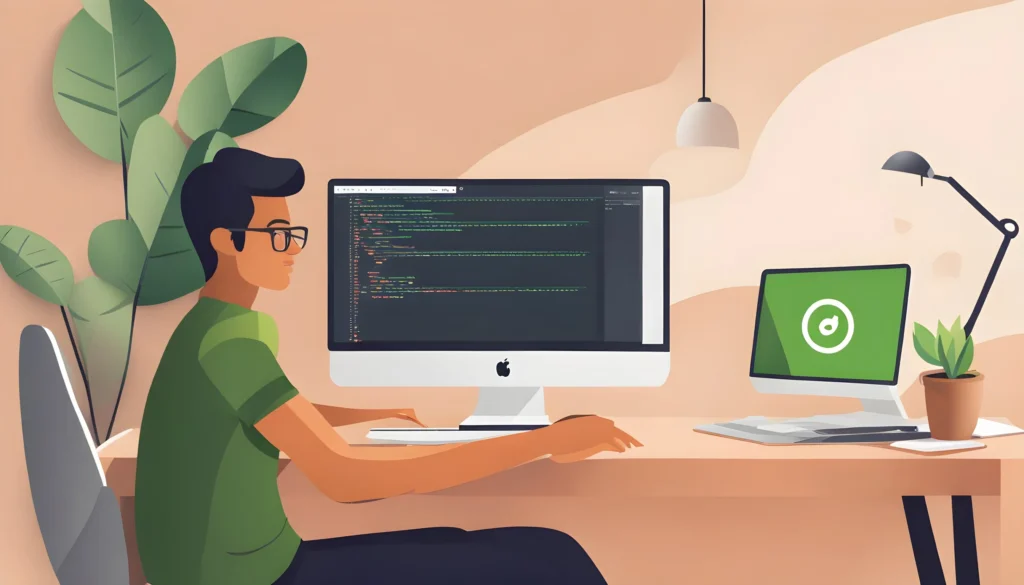
APIs running on Spring Boot are great, but once that traffic ramps up, latency and throughput can suffer. Your APIs may bog down, responses slow to a crawl, and servers max out. But have no fear – with a few simple tweaks using asynchronous programming, you can unlock hidden performance gains and keep your APIs blazing fast under load!
In this post, we’ll explore how adding a dash of @Async and CompletableFuture to your Spring MVC controller methods can optimize thread usage, slash latency, and squeeze every last drop of power from your servers. Read on to become an async master!
Table of Contents
- Understanding Asynchronous Programming
- Setting Up a Spring Boot Project
- Unleash the Power of Async!
- Using the @Async Annotation
- Leveraging CompletableFuture
- Thread Pool Configuration
- Error Handling and Exception Management
- Monitoring and Testing
- Real-world Use Cases
- Conclusion
- Frequently Asked Questions (FAQs)
1. Understanding Asynchronous Programming
Asynchronous programming allows your application to perform tasks concurrently without blocking the main thread. This is particularly beneficial when dealing with time-consuming operations like I/O or external service calls. In a Spring application, you can use the @Async annotation to mark a method as asynchronous.
By introducing concurrency, your application can handle multiple tasks simultaneously, leading to improved responsiveness and performance. Asynchronous programming is especially valuable in scenarios where tasks can be executed independently and do not depend on each other’s results.
Blocking is So Last-Season
Traditional MVC controllers process requests synchronously on one thread at a time. This can lead to a queue of requests piling up while waiting for long-running tasks like external service calls to complete. The blocked threads aren’t doing any real work during this time – they are just idling away, unable to handle new requests.
Asynchronous programming flips the script by freeing up these stalled threads. Instead of waiting around, the thread returns immediately and tasks execute independently in the background. New requests can be continuously processed while existing ones wrap up their work.
2. Setting Up a Spring Boot Project
To begin, let’s set up a Spring Boot project. If you haven’t already, make sure you have Spring Boot installed. You can create a new project using Spring Initializr or your preferred IDE. Ensure you include the necessary dependencies for Spring Web and Spring Aspects.
@SpringBootApplication @EnableAsync public class SpringAsyncDemoApplication { public static void main(String[] args) { SpringApplication.run(SpringAsyncDemoApplication.class, args); } }
Here, we’ve enabled asynchronous support with @EnableAsync. This annotation tells Spring to look for methods annotated with @Async and execute them asynchronously.
3. Unleash the Power of Async!
Now let’s look at some real benefits of using asynchronous programming in Spring controllers:
3.1. Slash Latency
Synchronous requests are forced to wait around for blocking operations to finish before a response is sent. But with async, responses fire back immediately while backend work handles itself.
Say goodbye to frustrated users stuck waiting on a loading spinner!
3.2. Increase Throughput
Async allows the same threads to handle more concurrent requests by preventing wasteful blocking. Resources are utilized more efficiently, throughput increases, and more total work gets done.
It’s like a 20-items-or-less express lane for your APIs!
3.3. Optimize Thread Usage
Thread blocking isn’t just wasteful – it can cripple API performance. If all threads are tied up waiting, new requests are rejected. Async enables fewer threads to produce the same throughput.
Do more with less using the magic of async!
3.4. Scale Vertically
Because async code can handle more concurrent requests per thread, it allows you to scale vertically more easily. Just add more CPU power or memory to existing servers instead of adding more nodes.
Get bigger biceps for your backend with async!
3.5. Go Non-Blocking with CompletableFuture
CompletableFuture provides a straightforward API for composing async logic without sacrificing code readability. It keeps code synchronous-looking while executing asynchronously.
Your code stays lean and mean on its async protein diet!
Get Pumping with Async Actions
To leverage these benefits, look for CPU or IO-bound operations in your controllers that can be moved to async methods. These are things like:
- External service/API calls
- Background data processing/crunching
- Intensive calculations
- File I/O
- Image processing
- PDF generation
- Email/reporting
- Data synchronization
The more you can shift these blocking operations into asynchronous flows, the faster and more efficient your APIs will become!
4. Using the @Async Annotation
The @Async annotation is the cornerstone of asynchronous programming in Spring. You can use it to mark a method as asynchronous, allowing it to execute in a separate thread. This means that the calling thread can continue its execution without waiting for the asynchronous method to complete. Here’s an example:
@Service public class MyService { @Async public CompletableFuture<String> doSomethingAsynchronous() { // Your asynchronous logic here return CompletableFuture.completedFuture("Async operation completed!"); } }
In this example, the doSomethingAsynchronous method is marked as asynchronous using @Async. The method returns a CompletableFuture<String>, which represents a future result of the asynchronous computation. You can also use other return types, depending on your requirements.
5. Leveraging CompletableFuture
CompletableFuture is a powerful class that represents a future result of an asynchronous computation. It allows you to perform operations asynchronously and combine their results. This is particularly useful when you have multiple asynchronous tasks that you want to run in parallel and then aggregate their results.
Here’s an example demonstrating how to use CompletableFuture to execute two asynchronous tasks:
@Service public class MyService { @Async public CompletableFuture<String> doSomethingAsynchronous() { // Simulate a time-consuming operation try { Thread.sleep(1000); } catch (InterruptedException e) { // Handle the exception } return CompletableFuture.completedFuture("Async operation completed!"); } @Async public CompletableFuture<Integer> doAnotherTaskAsynchronously() { // Another asynchronous task // ... return CompletableFuture.completedFuture(42); } }
In this example, we have two asynchronous methods: doSomethingAsynchronous and doAnotherTaskAsynchronously. Each of these methods performs a different asynchronous task and returns a CompletableFuture with the result.
CompletableFuture is used for returning async results from completed tasks. It has handy methods like thenApply(), thenAccept(), and thenCompose() to react to success or errors in async work.
For example:
@Async public CompletableFuture<Response> callExternalService() { // Call external API return CompletableFuture.completedFuture(response); } public void asyncHandler() { callExternalService() .thenApply(response -> { // Process successful response }) .exceptionally(ex -> { // Handle errors }); }
This allows async methods to be chained together while keeping code sequential and deterministic.
6. Thread Pool Configuration
Properly configuring a thread pool is essential to avoid creating too many threads, which can lead to resource exhaustion and decreased performance. By default, Spring uses a SimpleAsyncTaskExecutor, which doesn’t limit the number of threads it creates. You can, and should, customize the thread pool settings to control the number of concurrent threads.
In your application.properties or application.yml, you can customize the thread pool settings:
spring: task: execution: pool: core-size: 5 max-size: 10 queue-capacity: 100
These settings control the core pool size, maximum pool size, and the queue capacity for tasks. It’s essential to tailor these values to your application’s specific requirements. For instance, if your application needs to handle a large number of concurrent tasks, you may need a larger thread pool.
7. Error Handling and Exception Management
Handling exceptions in asynchronous code can be challenging. Asynchronous methods execute in separate threads, and exceptions thrown in those threads are not directly propagated to the calling thread. Therefore, it’s crucial to handle exceptions within the asynchronous method and return them as results if necessary.
@Async public CompletableFuture<String> doSomethingAsynchronous() { try { // Asynchronous logic } catch (Exception e) { // Handle the exception return CompletableFuture.failedFuture(e); } }
In this example, if an exception occurs during the execution of the asynchronous logic, it is caught, and the method returns a CompletableFuture containing the exception. The calling code can then handle the exception appropriately.
8. Monitoring and Testing
To ensure your API is performing optimally, use monitoring tools and conduct thorough testing. Measure the performance of your API both with and without asynchronous programming to determine its impact. Tools like Spring Boot Actuator can help you gather insights into your application’s performance.
By monitoring and testing, you can identify potential bottlenecks and areas that can benefit from asynchronous programming.
9. Real-world Use Cases
Let’s explore some real-world use cases where asynchronous programming can greatly benefit your Spring API:
1. Image Processing: When handling image uploads, processing images asynchronously can significantly reduce response times, especially if resizing or other transformations are involved.
2. External API Calls: If your API interacts with external services, using asynchronous calls can prevent your API from waiting for these services to respond, thereby improving overall responsiveness.
3. Batch Processing: When processing large data sets or performing batch operations, asynchronous programming can help distribute the work across multiple threads, making the process more efficient.
4. Chat Applications: In chat applications, handling messages asynchronously ensures that users can send and receive messages in real-time without blocking the system.
5. Notifications: Sending notifications, emails, or SMS messages can be done asynchronously, allowing the API to respond faster to user requests.
10. Conclusion
In conclusion, asynchronous programming using @Async and CompletableFuture in Spring is a powerful technique to enhance the performance and responsiveness of your API. By implementing it judiciously, configuring thread pools wisely, and effectively managing exceptions, you can take full advantage of these features to build high-performance Spring APIs that can handle a high load of concurrent requests.
As you embark on your journey to optimize your Spring APIs, remember that not all parts of your application may benefit equally from asynchronous programming. It’s essential to analyze your use cases and apply these techniques where they can make the most significant impact.
Now, take this knowledge and start optimizing your Spring APIs for better performance!
11. Frequently Asked Questions (FAQs)
Q1. When should I use asynchronous programming in my Spring API?
A. Asynchronous programming is beneficial when dealing with time-consuming tasks, such as I/O operations or external service calls. Use it selectively for specific parts of your API logic that can benefit from concurrency.
Q2. How do I choose the optimal thread pool configuration?
A. The thread pool configuration depends on your application’s needs. You can adjust the core size, maximum size, and queue capacity to balance resource utilization and responsiveness.
Q3. What’s the best way to handle exceptions in asynchronous code?
A. Handle exceptions within the asynchronous method, and make use of CompletableFuture.failedFuture()
to return errors as results.
Q4. Are there any performance trade-offs when using asynchronous programming?
A. While asynchronous programming can improve performance, it may introduce complexity and require careful error handling. Measure and test to determine the benefits in your specific use case.
For more insightful articles and in-depth guides on JAVA, Spring, Spring Boot and related topics, visit our homepage today.